In the fast-paced evolution of artificial intelligence (AI), transparency and trust are critical. Machine learning models often act as “black boxes,” making decisions without clearly explaining why. SHAP (SHapley Additive exPlanations) addresses this issue by providing explanations based on game theory, attributing specific feature contributions to individual predictions. This article walks through a hands-on example using SHAP to make machine learning models more interpretable and trustworthy.
What is SHAP?
SHAP uses Shapley values from cooperative game theory to attribute a value (or importance) to each feature in a model, helping us understand how features impact a model’s predictions. This method provides a consistent and interpretable measure of feature importance, making it easier to understand model behavior at the individual prediction level.
Tutorial Overview
This tutorial guides you through the following steps to use SHAP with a simple machine learning model:
- Data Generation and Preprocessing: We create a synthetic dataset to mimic house price data, with features like square footage, number of bedrooms, and house age.
- Model Training: We use a Random Forest model to predict house prices based on the generated features.
- SHAP Values Calculation and Visualization: SHAP is used to compute and visualize feature contributions, offering insights into how each feature impacts predictions.
Let’s dive into each step.
Step 1: Data Generation and Preprocessing
The first step is creating a synthetic dataset for house prices. This includes attributes such as square footage, the number of bedrooms, and the age of the house.
import pandas as pd import numpy as np # Generate synthetic data np.random.seed(42) data = pd.DataFrame({ 'sqft': np.random.randint(500, 3500, 100), 'bedrooms': np.random.randint(1, 5, 100), 'age': np.random.randint(0, 50, 100), 'price': np.random.randint(50000, 400000, 100) }) # Display the first few rows of data print(data.head())
This code generates a small, random dataset to simulate house characteristics and prices.
Step 2: Model Training
With the dataset ready, we proceed to train a Random Forest model to predict house prices.
from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestRegressor # Define features and target X = data[['sqft', 'bedrooms', 'age']] y = data['price'] # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Train the Random Forest model model = RandomForestRegressor(n_estimators=100, random_state=42) model.fit(X_train, y_train) # Check model performance score = model.score(X_test, y_test) print(f'Model R^2 Score: {score:.2f}')
In this step, we define our feature variables (sqft
, bedrooms
, age
) and target variable (price
). After splitting the data into training and test sets, we fit a Random Forest model to predict house prices based on the features.
Step 3: Calculating and Visualizing SHAP Values
With the model trained, we can now use SHAP to calculate and visualize feature importance for individual predictions. This allows us to see how each feature impacts the model’s predictions.
Install SHAP
If SHAP isn’t installed, run:
pip install shap
Calculating SHAP Values
# Choose a specific instance to explain instance_index = 0 shap.force_plot(explainer.expected_value, shap_values[instance_index, :], X_test.iloc[instance_index, :])
The force_plot
function displays the SHAP values for a specific instance, showing how each feature impacts the prediction for that single instance.
Why SHAP?
By using SHAP, you gain a clearer understanding of how each feature affects predictions. This transparency is invaluable, especially in fields where understanding model behavior is critical, such as healthcare, finance, and law. SHAP provides a means to establish trust and accountability in machine learning applications.
Conclusion
SHAP is a powerful tool for explainable AI, making machine learning models more interpretable and transparent. This tutorial introduced SHAP, from setting up a model to visualizing feature contributions for predictions. By integrating SHAP into your projects, you can foster greater transparency, understanding, and trust in your AI models.
For further details, check out my complete tutorial on GitHub. Experiment with the code, and consider adapting it to your specific needs. Embracing explainability is a step towards responsible and transparent AI.
Also, check my article on LinkedIn titled “Explainable AI: Trust and Transparency with SHAP” which is part of the GnoelixiAI Hub Newlsetter.
Read Also:
- Understanding Artificial Intelligence: A Human-Centric Overview
- Understanding Machine Learning: The Heart of Modern AI
- Addressing AI Risks: Achieving the AI Risk Management Professional Certification
- Mastering Scaled Scrum: Earning the Scaled Scrum Professional Certification
- Strengthening Agile Leadership: Achieving the Scrum Master Professional Certificate
- Advancing My Expertise in AI: Earning the CAIEC Certification
- Achieving the CAIPC Certification: Advancing My AI Expertise
Subscribe to the GnoelixiAI Hub newsletter on LinkedIn and stay up to date with the latest AI news and trends.
Subscribe to my YouTube channel.
Reference: aartemiou.com (https://www.aartemiou.com)
© Artemakis Artemiou
Rate this article:
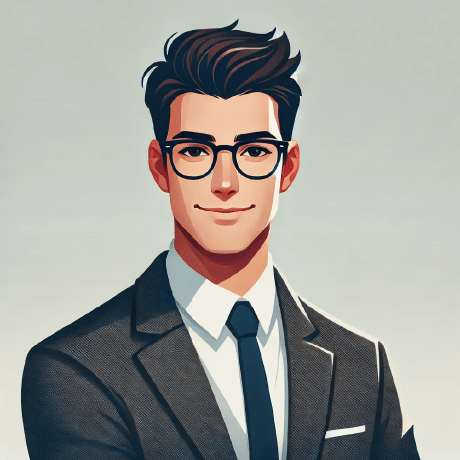
Artemakis Artemiou is a seasoned Senior Database and AI/Automation Architect with over 20 years of expertise in the IT industry. As a Certified Database, Cloud, and AI professional, he has been recognized as a thought leader, earning the prestigious Microsoft Data Platform MVP title for nine consecutive years (2009-2018). Driven by a passion for simplifying complex topics, Artemakis shares his expertise through articles, online courses, and speaking engagements. He empowers professionals around the globe to excel in Databases, Cloud, AI, Automation, and Software Development. Committed to innovation and education, Artemakis strives to make technology accessible and impactful for everyone.