Hi friends! Today, let’s explore how core Python data structures form the bedrock of AI and machine learning workflows. While we’ll cover the usual suspects—lists, tuples, dictionaries, and sets—we’ll also discuss how these constructs streamline data preprocessing, feature engineering, and model input pipelines. Whether you’re orchestrating a deep learning model or just tinkering with a simple classifier, the right data structures can make your AI-driven projects far more efficient and effective.
What is a Data Structure?
A data structure is essentially a container that allows you to store, manage, and organize data. Every programming language provides its own set of data structures, which serve as the building blocks for working with any form of data. In Python, these versatile tools aren’t just for everyday scripting—when you’re dealing with massive datasets, complex transformations, and intricate model architectures, knowing how to leverage the right data structures can be a game-changer in the AI domain.
Main Python Data Structures
Below we’ll examine some of Python’s most commonly used non-primitive data structures. These structures often underpin tasks like cleaning raw data, creating feature sets, and batch-feeding inputs into machine learning models. While this list is not exhaustive, it’s a great starting point, especially if you’re aiming to craft AI-driven applications that are both scalable and maintainable.
List
A List in Python allows you to store multiple values in a single variable. Lists are mutable, meaning you can easily update their contents at runtime. This flexibility is crucial in AI development, where you might load initial datasets into lists, then dynamically augment or shuffle them for training and validation workflows.
# List Example in Python # Define List vehiclesList = ["car", "motorbike", "train"] # Print Full List print(vehiclesList) # Print a Specific Element from the List print(vehiclesList[0])
In AI projects, you might use lists to hold a collection of training samples, image file paths, or tokenized text, giving you quick index-based access to tweak, transform, and sample your data as needed.
# Tuple Example in Python # Define Tuple vehiclesTuple = ("car", "motorbike", "train") # Print Full Tuple print(vehiclesTuple) # Print a Specific Element from the Tuple print(vehiclesTuple[0])
If you’re working on a machine learning experiment, a tuple could hold a combination of hyperparameters (e.g., learning rate, batch size), helping you maintain a stable, unchanging reference set during training runs.
Dictionaries
A Dictionary stores data in key-value pairs. This is incredibly useful in AI workflows, where you might map feature names to values, labels to class indices, or configuration options to specific parameters. With dictionaries, you can quickly access the exact piece of information you need, making feature engineering and data transformations more intuitive.
Example:
# Dictionary Example in Python # Define Dictionary vehiclesDictionary = {"Type": "Car", "Color": "Red"} # Print Dictionary print(vehiclesDictionary)
In AI contexts, dictionaries can store model performance metrics keyed by epoch, class distributions keyed by labels, or configuration parameters keyed by descriptive names. This seamless organization speeds up debugging and fine-tuning.
Sets
A Set in Python holds unique, unordered, and immutable elements. Sets are perfect for managing large collections of unique tokens, classes, or feature categories—critical tasks in AI-driven natural language processing (NLP) or classification workflows.
Example:
# Set Example in Python # Define Set vehiclesSet = {"car", "motorbike", "train", "train"} # Print Set print(vehiclesSet)
If you run the above code, you’ll notice the duplicate “train” entry is removed automatically. In an AI setting, sets help you ensure that your training vocabulary remains clean and free of duplicates, making it simpler to handle downstream tokenization, encoding, or clustering operations.
Beyond Collections: Other Python Data Structures
Python also provides primitive data structures like integers, floats, strings, and Booleans. While these often serve as building blocks, think of them as the atoms from which you construct more complex AI artifacts. Combined with lists, tuples, dictionaries, and sets, they empower you to orchestrate complex workflows—such as normalizing numeric inputs for neural networks or encoding labels as strings before converting them into integer indices.
Putting It All Together in an AI Context
When building AI applications, whether it’s a sentiment analysis model in NLP, a computer vision classifier, or a forecasting system, these Python data structures help you streamline data ingestion and transformation. For instance, you might load your raw dataset into lists, use tuples for fixed hyperparameter sets, employ dictionaries to map between label classes and IDs, and leverage sets to ensure your feature inputs remain clean and distinct. Understanding these tools is essential for creating efficient data pipelines that can scale as your project grows.
Learn to Integrate Python and SQL Server for AI Workflows
For even deeper capabilities, check out my course, “Working with Python on Windows and SQL Server Databases.” This will help you create robust data pipelines that feed into your machine learning models. By interacting with SQL Server, you can store training data, query large datasets, and seamlessly integrate with Python-based AI frameworks, ensuring you have the right data structure for every step of your AI journey.
By the end of this course, you will know how to:
- Install Python on Windows and set up your development environment with Visual Studio Code and the proper extensions.
- Connect Python applications to SQL Server instances and databases.
- Execute SELECT, INSERT, UPDATE, and DELETE T-SQL statements directly from Python code.
- Work with SQL Server DMVs, functions, stored procedures, and handle parameters and exceptions.
- Use these operations to fuel your AI models with structured, high-quality training data.
Read Also:
- Advancing My Expertise in AI: Earning the CAIEC Certification
- Achieving the CAIPC Certification: Advancing My AI Expertise
- Understanding Artificial Intelligence: A Human-Centric Overview
- Addressing AI Risks: Achieving the AI Risk Management Professional Certification
- Mastering Scaled Scrum: Earning the Scaled Scrum Professional Certification
- Strengthening Agile Leadership: Achieving the Scrum Master Professional Certificate
- Advancing My Expertise in AI: Earning the CAIEC Certification
- Achieving the CAIPC Certification: Advancing My AI Expertise
Subscribe to the GnoelixiAI Hub newsletter on LinkedIn and stay up to date with the latest AI news and trends.
Subscribe to my YouTube channel.
Reference: aartemiou.com (https://www.aartemiou.com)
© Artemakis Artemiou
Rate this article:
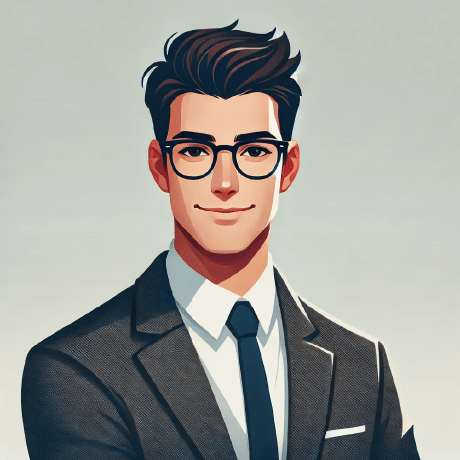
Artemakis Artemiou is a seasoned Senior Database and AI/Automation Architect with over 20 years of expertise in the IT industry. As a Certified Database, Cloud, and AI professional, he has been recognized as a thought leader, earning the prestigious Microsoft Data Platform MVP title for nine consecutive years (2009-2018). Driven by a passion for simplifying complex topics, Artemakis shares his expertise through articles, online courses, and speaking engagements. He empowers professionals around the globe to excel in Databases, Cloud, AI, Automation, and Software Development. Committed to innovation and education, Artemakis strives to make technology accessible and impactful for everyone.